Testsigma’s API Testing Guide.
Functional Testing: Validating Core Behavior
Functional testing checks if your API works as designed and documented. This involves systematically testing endpoints with different inputs and verifying the outputs match specifications.
Key aspects to test include:
- Response Status Codes: Verify that endpoints return correct HTTP status codes (200, 400, 500 etc.) for various scenarios
- Response Data: Check that returned data matches expected format, types and structure
- Error Handling: Test with invalid inputs and edge cases to ensure proper error messages and graceful failure handling
Integration Testing: Checking System Connections
Most APIs need to work with other services and systems. Integration testing confirms these connections function properly and data flows correctly between components.
Focus areas include:
- Multi-Endpoint Workflows: Test sequences of API calls that work together to complete business processes
- External Dependencies: Validate interactions with databases, third-party services and other integrated systems
Performance testing helps identify bottlenecks and ensure your API can handle expected load. This prevents issues before they impact users.
Key test types:
- Load Testing: Measure response times and error rates under normal and peak traffic
- Stress Testing: Find breaking points by gradually increasing load beyond normal limits
- Duration Testing: Run sustained tests over many hours to catch memory leaks and degradation
Security Testing: Finding Vulnerabilities
Security testing helps protect your API from attacks and unauthorized access. This is critical for maintaining data safety and user trust.
Essential security tests:
- Access Controls: Verify that authentication and authorization properly restrict access to endpoints
- Input Safety: Test resistance to injection attacks and malicious inputs
- Data Protection: Confirm proper encryption of sensitive data in transit and storage By implementing these core testing practices, teams can build APIs that are reliable, performant and secure. This structured approach helps catch issues early and ensures APIs work properly in production environments.
Building Bulletproof Security Testing
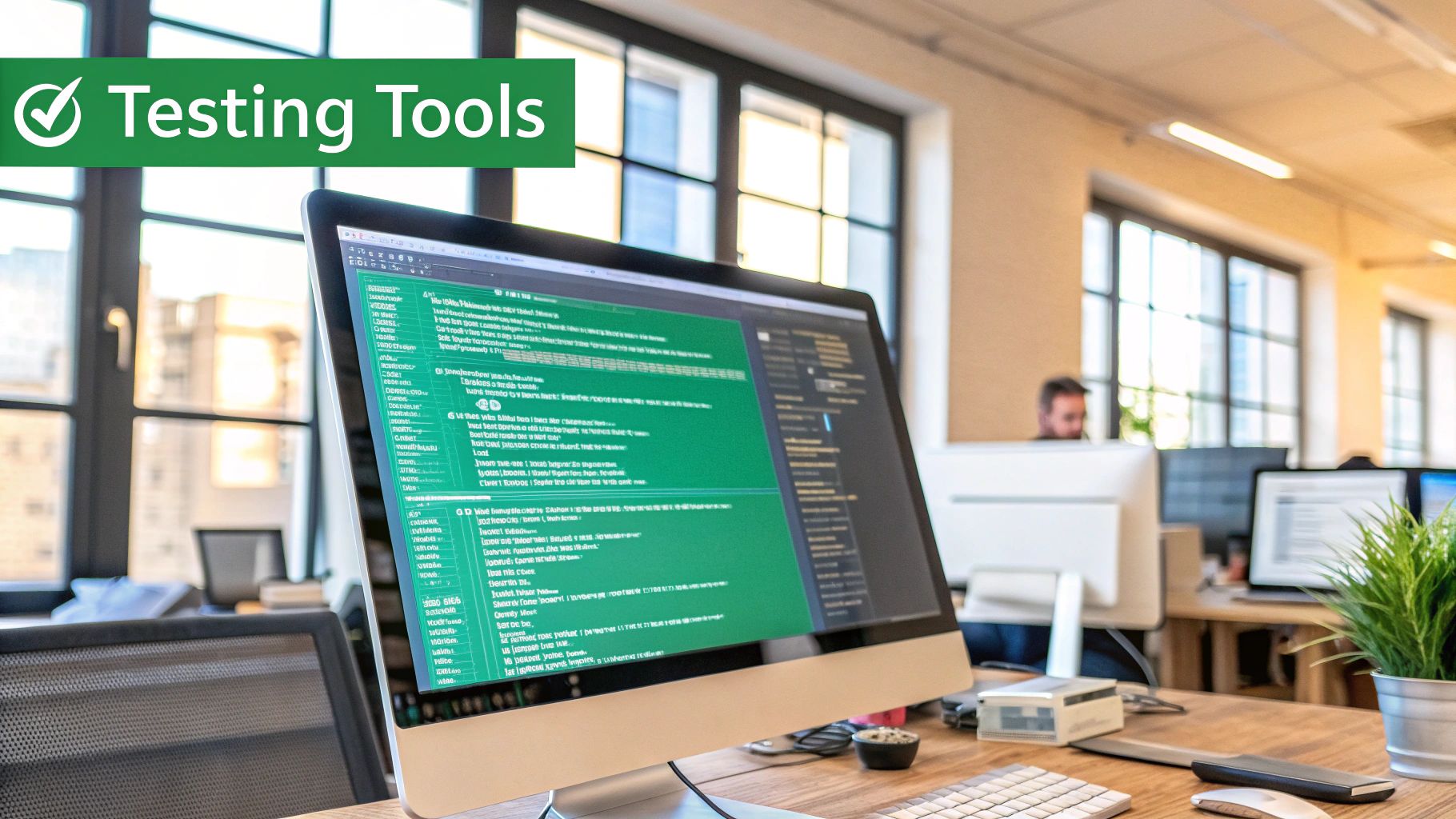
Strong security testing is essential for any API. Understanding how to properly test your API’s security helps prevent vulnerabilities and maintains user trust. The stakes are high - a single API flaw can have serious consequences. Take the 2017 Equifax breach for example, where an API vulnerability exposed sensitive data of over 147 million people. Learn more about protecting your API through comprehensive testing.
Authentication and Authorization
Every API needs robust user verification. Authentication confirms who users are, while authorization controls what they can access. Testing these systems thoroughly helps prevent unauthorized access and data breaches.
- Test Authentication: Check different login methods like API keys, OAuth tokens, and JWTs. Make sure they work correctly and resist common attacks.
- Validate Authorization: Test user permissions and roles carefully. Look for ways users might bypass restrictions to access protected data.
Data Protection and Validation
Your API must protect sensitive information both during transmission and storage. Input validation is equally important to prevent malicious attacks.
- Encryption in Transit: Use HTTPS and proper TLS/SSL settings for all API communication
- Data at Rest Security: Encrypt stored sensitive data properly. Follow relevant standards like GDPR and HIPAA
- Input Validation: Check all user input thoroughly to stop injection attacks. Test with various malicious inputs to verify proper sanitization
Business Logic Vulnerabilities
Some security issues come from flaws in how the API works, not just technical problems. These require careful testing of API behavior and workflows.
- Scenario-Based Testing: Create real-world test cases to find potential weak points in API processes
- Negative Testing: Use incorrect or unexpected inputs to ensure proper error handling without exposing sensitive details
Integrating Security into the CI/CD Pipeline
Add security checks to your development pipeline to catch issues early and often.
- Automated Security Scans: Use security tools to find common problems during development
- Security Regression Testing: Run security tests with each update to prevent old issues from returning
Establishing Security Benchmarks
Set clear security goals and standards for your API. Define acceptable risk levels and regularly check security status. Review and update these standards as your API grows. Regular testing and updates help prevent vulnerabilities before they become problems. Following these practices helps build a secure and reliable API.
Performance testing is essential to verify if your API can handle real user demands. This goes beyond basic functionality checks to examine how well your API performs under pressure. Running tests that reflect actual usage helps find hidden performance issues and confirms your API stays stable during high-traffic periods.
Different types of performance tests check various aspects of API stability. Here are the key testing approaches you should consider:
- Load Testing: Simulates normal traffic levels to check API response under everyday conditions. This creates a baseline for expected performance and spots potential issues.
- Stress Testing: Tests API behavior at extreme loads to find breaking points. This helps determine maximum capacity and plan for growth.
- Endurance Testing: Runs tests over long time periods to catch issues like memory leaks and slow performance decline. This verifies your API maintains stability during extended use.
Good performance tests need to match real usage patterns to give useful results. Consider these key factors:
- User Behavior Simulation: Create tests that copy actual user actions. Include different request types, parameters, and data sizes that match real scenarios.
- Traffic Modeling: Study past usage data to map out expected traffic patterns. Build these patterns into your tests to check how your API handles busy periods.
- Environment Replication: Use a test environment that matches your production setup. Server specs, network settings, and database configuration should be similar. Performance testing becomes critical for high-traffic APIs. For example, if an API needs to handle 500 requests per hour with 0.01 second response times, testing helps find limits. In real cases, when traffic hits 1200 requests per hour, performance might drop. Regular testing helps measure performance and prepare for growth. Learn more details about API testing at Software Testing Help.
Interpreting Results and Optimization
After running performance tests, analyzing the data helps guide improvements:
- Find Bottlenecks: Check response times, errors, and resource usage to spot problems. Look at database queries, network delays, and code performance.
- Set Performance Standards: Regular testing creates benchmarks to track changes. This helps catch performance drops and measure how updates affect speed.
- Make Data-Based Improvements: Use test results to focus optimization work. This might mean improving code, tuning databases, or adding more servers. These testing methods give developers clear insights into API performance under real conditions. This helps create reliable APIs that work well at scale. Tools like DocuWriter.ai can help by handling documentation tasks, letting developers focus on performance improvements.
Avoiding Common Testing Pitfalls
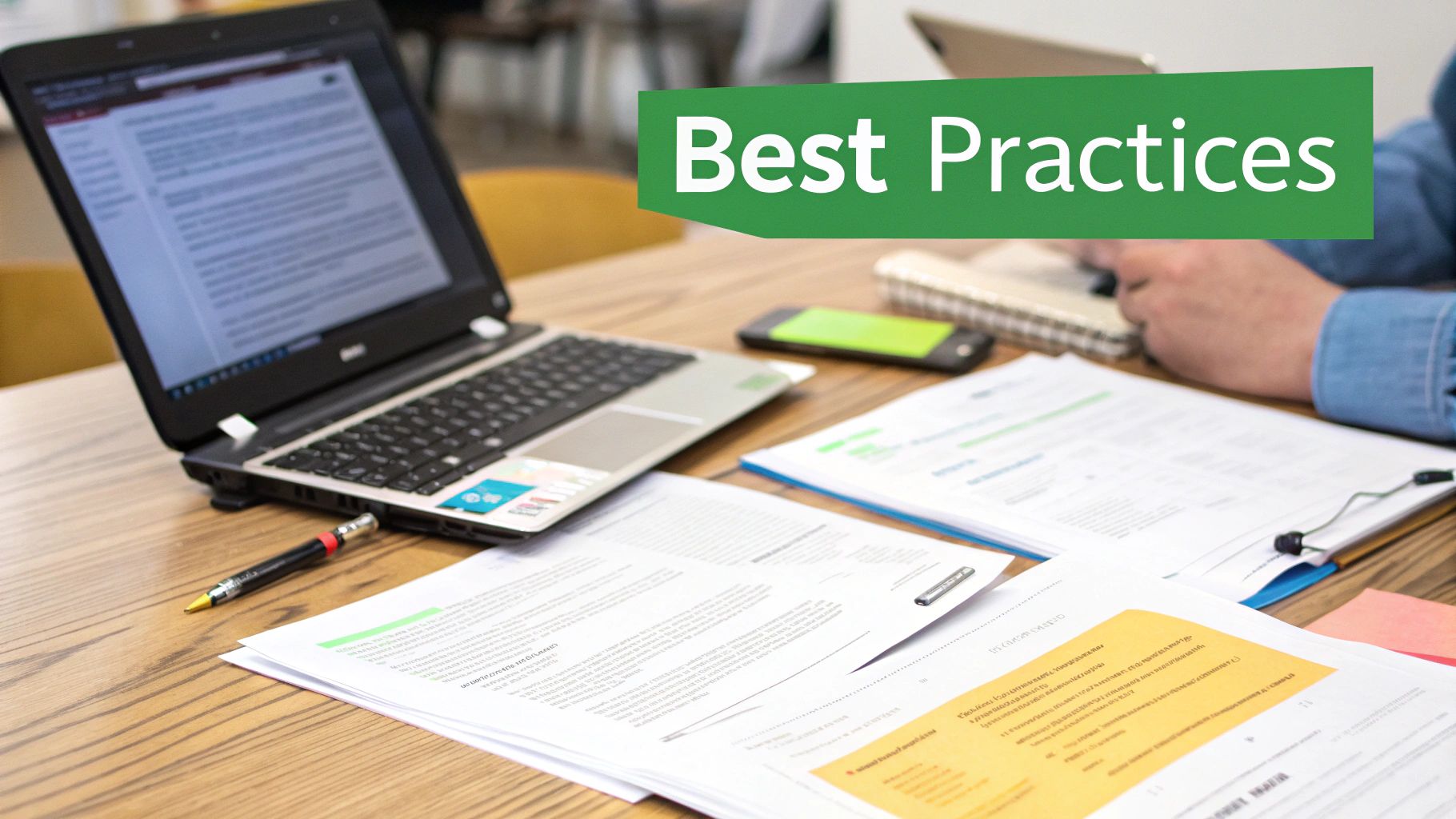
A strong testing strategy is essential, but certain mistakes can seriously impact your API testing effectiveness. Let’s explore the key pitfalls to watch out for and practical ways to avoid them.
Neglecting Negative Testing
Most testing guides focus on checking if APIs work correctly with valid inputs. However, negative testing - deliberately using invalid inputs - is equally important. When you test error handling, you ensure your API responds appropriately to problems instead of crashing. For example, sending text data to a numbers-only endpoint should return a clear error message rather than failing unexpectedly.
Inadequate Test Coverage
Good testing means checking many different scenarios, especially edge cases. Missing key test cases can hide serious issues. For instance, if your API accepts numbers between 1-100, make sure to test:
- The minimum value (1)
- The maximum value (100)
- Invalid values just outside the range (0 and 101)
- Random valid values in between
Overlooking Environment Differences
When your test environment doesn’t match production, test results become unreliable. A common example is using different database settings between testing and production environments. To get accurate results, keep your test setup as close as possible to the real production environment.
Poor Test Data Management