Understanding CI/CD Pipeline Fundamentals
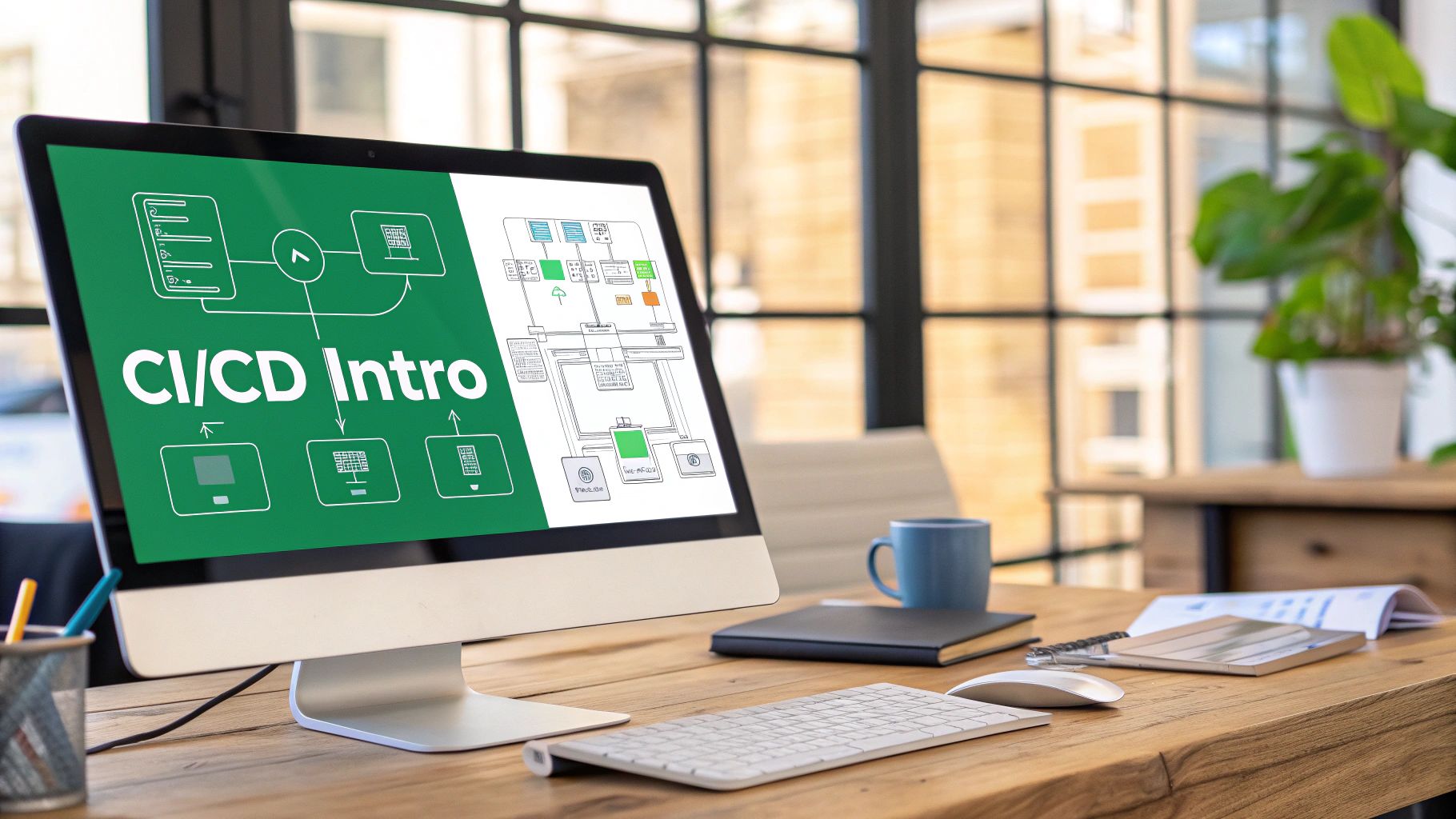
A CI/CD pipeline forms the core of modern software development practices. It empowers teams to deliver high-quality code rapidly and reliably. This exploration delves into the fundamental concepts of CI/CD and how they contribute to a more efficient development process. Grasping these principles is key for anyone seeking to implement or enhance their CI/CD workflows.
What Is Continuous Integration (CI)?
Continuous Integration (CI) is the practice of merging code changes frequently into a shared repository. Automated builds and tests are triggered after each merge. This contrasts with traditional development where developers might integrate their work less often, leading to larger, more complex merges. CI helps catch and address integration issues early.
This early integration approach minimizes the risk of major conflicts arising later in the development cycle. It ultimately contributes to a smoother, more efficient workflow.
What Is Continuous Delivery (CD)?
Building upon the foundation of CI, Continuous Delivery (CD) automates the software release process. Every code change that successfully passes the CI stage is automatically deployed to a staging environment. This staging environment allows for more comprehensive testing before release.
CD ensures the software is always ready for deployment with minimal manual intervention. This allows teams to respond swiftly to market changes and user feedback. Frequent releases become a standard practice, enabling faster iteration cycles.
What Is Continuous Deployment?
Continuous Deployment often gets confused with Continuous Delivery, but it takes automation a step further. In a Continuous Deployment setup, every change that clears all testing phases is automatically deployed to production. No manual approvals are necessary.
This accelerates the feedback loop, leading to continuous improvement. However, this approach requires a high level of trust in the automated testing and deployment procedures. Robust and reliable automated systems are crucial for success.
Key Components of a CI/CD Pipeline
High-performing CI/CD pipelines typically incorporate these essential components:
- Version Control: A system like Git is crucial for managing code changes and facilitating developer collaboration. It provides a central repository to track code versions and resolve merging conflicts efficiently.
- Build Automation: Tools such as Maven or Gradle automate the process of compiling code, executing tests, and packaging the application. This ensures consistent and reproducible builds across different environments.
- Automated Testing: Automated tests, including unit, integration, and end-to-end tests, are essential for verifying the quality and functionality of the code. These tests form the backbone of a reliable CI/CD pipeline.
- Deployment Automation: Tools like Ansible or Kubernetes automate the deployment process. This minimizes the risk of human error and facilitates rapid deployments to various environments. CI/CD adoption has increased significantly in recent years. Many development teams now prioritize integrating security measures throughout their pipelines. These measures include static code analysis, vulnerability scanning, and security testing within the CI/CD process itself. You can delve deeper into CI/CD security trends at Oshyn. This focus on integrated security is paramount for delivering secure and reliable software. With the growing importance of automation and speed in software development, understanding CI/CD fundamentals is essential for efficient and high-quality software delivery.
Building Your First CI/CD Pipeline
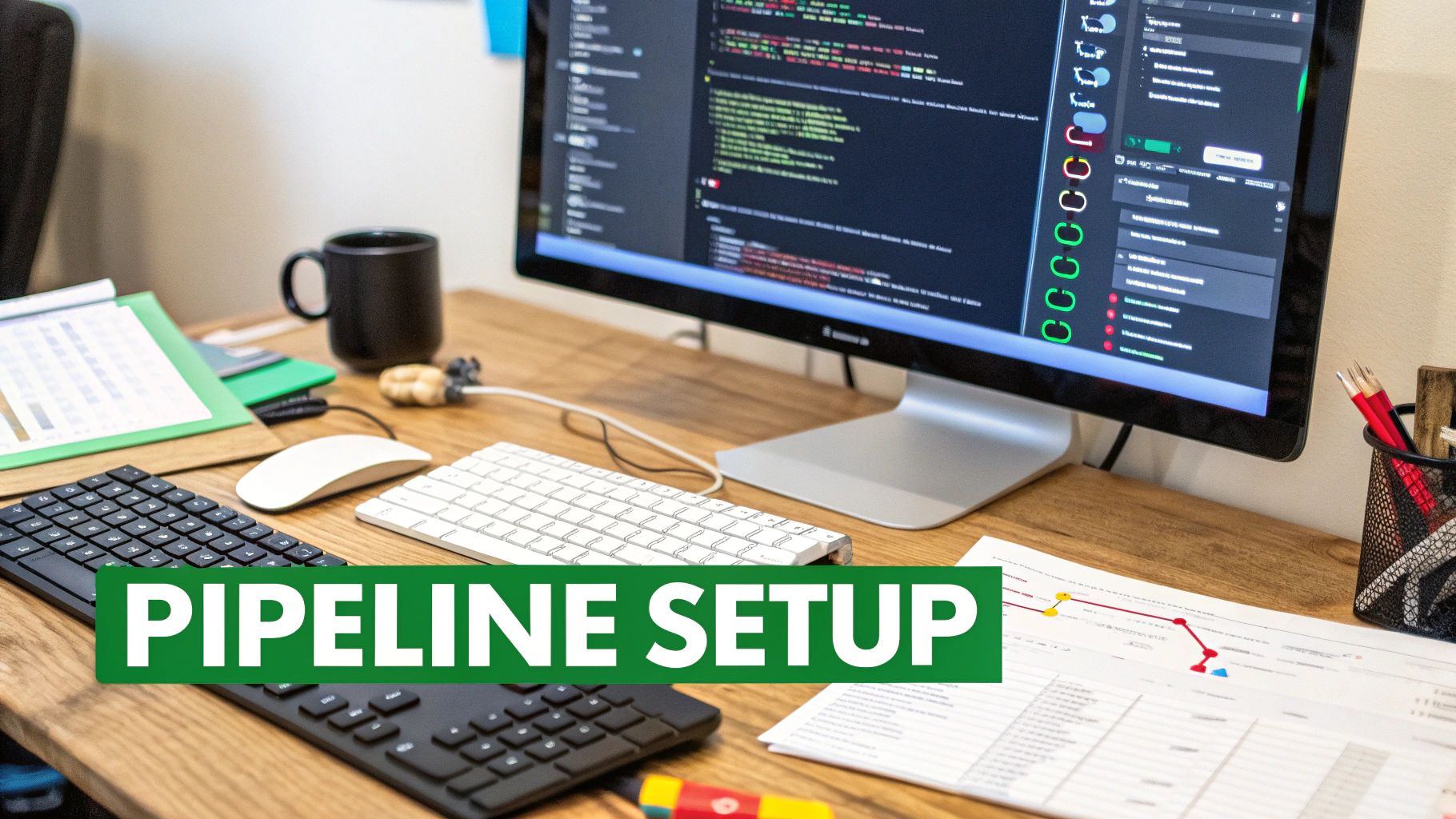
Let’s dive into the practical side of Continuous Integration/Continuous Delivery (CI/CD). This guide provides a step-by-step approach to building your first CI/CD pipeline. We’ll explore tool selection, environment setup, and pipeline scripting, giving you a strong foundation for automation.
The success of your CI/CD pipeline hinges on choosing the right tools. The ideal choice depends on various factors, including project size, team experience, and specific needs.
- Jenkins: Jenkins is a highly adaptable open-source automation server. Its extensive plugin support makes it a versatile choice for many projects.
- GitLab CI/CD: Tightly integrated within the GitLab platform, this tool streamlines version control and CI/CD workflows, simplifying collaboration.
- GitHub Actions: Built directly into GitHub repositories, GitHub Actions offers seamless workflow automation, especially convenient for GitHub-hosted projects.
- CircleCI: This cloud-based CI/CD platform, CircleCI, is known for its scalability and user-friendly interface. Its cloud-native design allows for quick setup.
- Travis CI: Travis CI is another cloud-based solution appreciated for its simplicity and broad language support. It’s a great option for quick CI/CD implementation.
Setting Up Your Environment
Once you’ve chosen your CI/CD platform, the next step is environment configuration.
- Creating A Repository: Start by storing your project’s code in a version control system like Git. This creates a central, reliable codebase.
- Installing Necessary Tools: Install your chosen CI/CD tool and any dependencies on your build server or local machine. Proper configuration is essential for smooth operation.
- Configuring Access Credentials: Securely manage access between your CI/CD platform and your code repository. Prioritize secure storage and access control for sensitive information.
Writing Your CI/CD Pipeline Configuration
The heart of your CI/CD pipeline is its configuration file. This file dictates the steps executed during the CI/CD process.
- Build: This stage compiles and packages your application, preparing it for deployment.
- Test: Thorough testing, from unit tests to integration tests, ensures code quality. This stage is vital for catching potential issues early.
- Deploy: Automate deployment to your staging and production environments. This streamlines the release process and minimizes errors. A basic configuration example in YAML:
stages:
- build
- test
- deploy build: stage: build script:
- mvn clean install
test: stage: test script:
deploy: stage: deploy script:
This simplified example illustrates the structure and workflow. Real-world pipelines are often more intricate. Adapting to your project’s specific needs is key. Implementing a CI/CD pipeline can dramatically improve developer productivity and even revenue. Some companies have seen a 30% increase in developer productivity after implementing CI/CD. This gain comes from automating tasks, allowing developers to focus on coding. For more on the impact of CI/CD, see the DevOps Institute. Building your first pipeline can seem challenging, but these steps will get you started with automated software delivery. This more efficient process allows you to develop, test, and deploy code faster and with more confidence.
Mastering Automated Testing In Your CI/CD Workflow
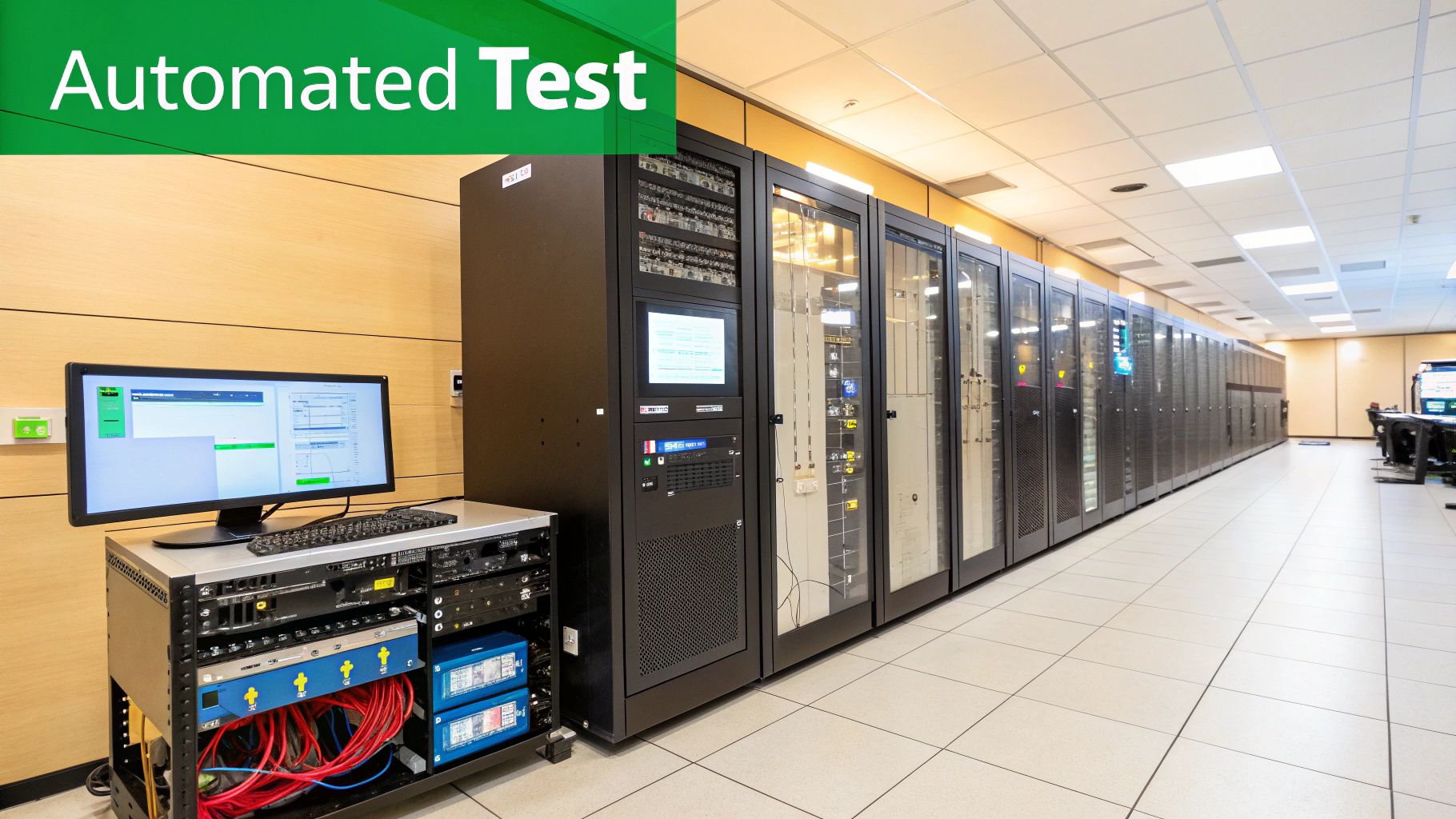
Automated testing is essential for a successful CI/CD pipeline. It helps maintain high code quality and prevents bugs from impacting users. This section explores practical strategies for integrating automated tests seamlessly into your workflow, resulting in robust and reliable software delivery.
Implementing Different Testing Layers
Effective testing strategies utilize multiple layers, each focusing on specific aspects of the application. This layered approach provides comprehensive coverage and early bug detection.
- Unit Tests: These tests isolate individual components or functions to verify their behavior. Unit tests are typically quick to execute and excellent for catching issues early in the development process. For a deeper dive into unit testing, check out this guide: How to master unit testing.
- Integration Tests: Once individual units are verified, integration tests examine how different components interact. They ensure that the various parts of the system work together harmoniously.
- End-to-End (E2E) Tests: E2E tests simulate real user scenarios, evaluating the entire application flow. These tests provide valuable feedback on the user experience and help identify potential issues in the complete system. To further illustrate these different testing approaches and their place within a CI/CD pipeline, let’s examine the following comparison:
Common Test Types in CI/CD Pipelines: A Comparison of different testing approaches and their implementation in CI/CD workflows
As shown in the table, each test type plays a distinct role in ensuring overall software quality. Unit tests offer quick feedback during development, while E2E tests provide a final check of the entire system before release. Integration tests bridge the gap, ensuring that individual components function correctly together.
Structuring Tests for Meaningful Feedback
Tests should provide clear, actionable results. Minimizing false positives is essential for maintaining confidence in the testing process.
- Clear Assertions: Use specific assertions to validate expected outcomes. This precision helps pinpoint the exact location of problems when tests fail.
- Representative Test Data: Use realistic test data that mirrors real-world usage patterns. This ensures tests cover a wide range of scenarios, including common use cases and edge cases.
Organizing Test Suites for Maximum Efficiency
Strategic organization of test suites is crucial for optimizing pipeline speed and efficiency.
- Prioritize Fast Tests: Execute quick tests like unit tests early. This provides rapid feedback during development.
- Parallelize Test Execution: Run tests concurrently whenever possible. This significantly reduces the overall testing time, particularly in larger projects.
Handling Test Data and Flaky Tests
Proper data management and handling unreliable tests are vital for maintaining test integrity.
- Test Data Management: Implement effective strategies to manage test data. Consider techniques like mocking or test doubles to isolate components and ensure consistent inputs.
- Managing Flaky Tests: Address flaky tests—tests that yield inconsistent results—promptly. These tests reduce confidence in the suite. Quarantining or fixing them improves the overall reliability of your tests. By adopting a multi-layered testing approach, structuring tests for clarity, and effectively managing test data, you can build a robust CI/CD pipeline. This delivers high-quality software consistently, catching bugs early and preventing them from reaching production. This approach saves time and resources while enhancing customer satisfaction.
Deployment Strategies That Minimize Risk
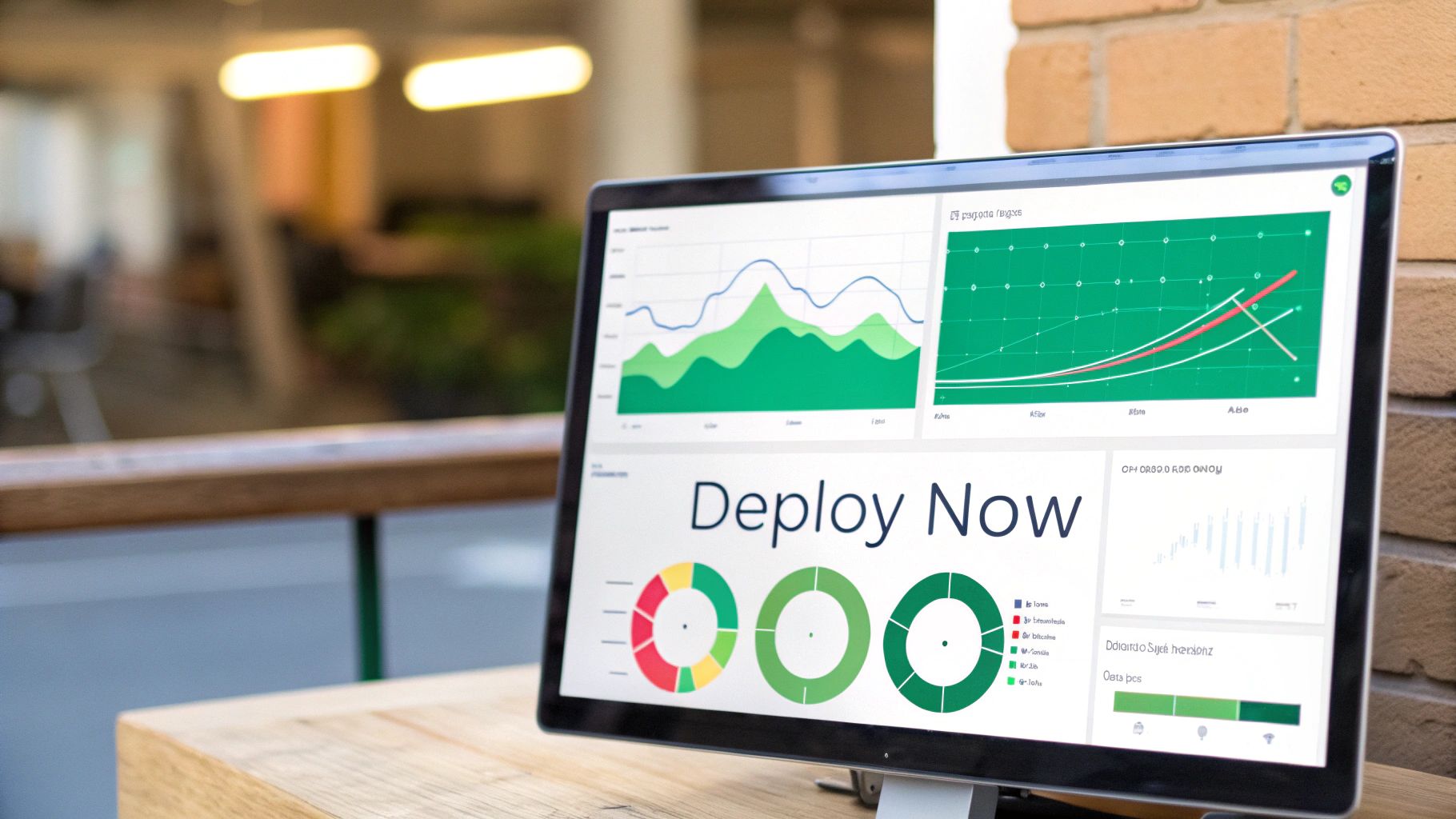
Automated testing helps verify the quality of your code. But deploying it effectively is just as important. This involves choosing the right deployment strategy and having solid rollback mechanisms. Let’s explore some strategies that minimize risk and maximize efficiency, allowing for faster feedback and reducing the impact of potential issues.
Blue-Green Deployments
Blue-green deployments use two identical environments: “blue” (live) and “green” (staging). New code goes to the green environment, gets thoroughly tested, and then traffic switches over from blue to green. This makes green the live environment.
This minimizes downtime and offers a quick rollback. If problems occur, just switch traffic back to blue. This ensures minimal disruption for users during updates, with seamless transitions and a simplified rollback process. Blue-green deployments are a safe and efficient way to manage releases.
Canary Releases
Canary releases involve deploying new code to a small group of users. This lets you monitor performance in a real-world setting before a full rollout. This “canary” group acts like a testbed, revealing any bugs or performance issues with real users.
If the canary release is successful, the new version gradually rolls out to everyone else. This approach minimizes the impact of potential problems by allowing real-world testing before full deployment. Canary releases are vital for verifying code stability under real usage patterns.
Feature Flags
Feature flags let you enable or disable features in production without redeploying the whole application. This allows for targeted testing and phased rollouts. You can control who sees a new feature by assigning users or groups to the flag.
This granular control lets teams experiment with new functions and gather live user feedback. It provides a controlled way to test and roll out features to different user segments. Feature flags are powerful tools for managing releases and A/B testing different implementations.
Infrastructure as Code and Rollback Mechanisms
Robust infrastructure and clear rollback processes are often necessary for implementing these deployment strategies successfully.
- Infrastructure as Code (IaC): IaC defines your infrastructure in code, enabling automated provisioning and management of environments. This ensures consistent and reproducible deployments. For IaC best practices, check out: How to master Infrastructure as Code.
- Rollback Mechanisms: Essential for any deployment, rollback plans offer a fast way to revert to a previous stable version if errors occur. These mechanisms are key for minimizing downtime and user impact during unexpected problems.
Database Migrations and Environment-Specific Configurations
Two often-overlooked aspects of CI/CD pipelines are database migrations and environment-specific configurations. These are critical for smooth deployments.
- Database Migrations: Carefully plan and automate your database schema updates alongside your code deployments to ensure consistency between your application code and data storage.
- Environment-Specific Configurations and Secrets: Use appropriate tools and techniques to manage configurations and secrets securely across different environments. This prevents sensitive information exposure and ensures correct application behavior in each environment. Speaking of DevOps, a recent ‘State of CI/CD Report 2024’ found that 83% of developers are involved in DevOps activities. This highlights the increasing importance of DevOps in modern software development. The report also notes a learning curve, with less experienced developers adopting fewer DevOps practices. See more detailed statistics here: State of CI/CD 2024 Report. Implementing these strategies and considering these crucial aspects transforms your CI/CD pipeline into a tool for reliable software delivery, allowing for confident releases and faster feedback, leading to a more efficient and successful development process.
Automated deployments are a significant step towards efficient software delivery. However, simply having a CI/CD pipeline isn’t enough. To truly optimize your process, you need to measure its effectiveness. This involves tracking key metrics that provide insights into your pipeline’s performance and identifying areas for improvement. This article explores how high-performing teams use data-driven insights to enhance their CI/CD pipelines, focusing on metrics that directly impact delivery.
Tracking the right metrics allows you to understand your pipeline’s strengths and weaknesses, enabling data-driven improvements. Here are some of the most critical:
- Build Time: This measures the time taken to compile and package your application. Shorter build times translate directly to faster feedback and quicker iteration cycles. Reducing build time is often a top priority for optimizing the development process.
- Test Coverage: This indicates the percentage of your code covered by automated tests. Higher test coverage generally correlates with greater code quality and fewer bugs. Aiming for comprehensive test coverage helps ensure reliable software.